protected static String getBaseEnvLinkURL() { String baseEnvLinkURL=null; HttpServletRequest currentRequest = ((ServletRequestAttributes)RequestContextHolder. currentRequestAttributes()).getRequest(); // lazy about determining protocol but can be done too baseEnvLinkURL = "http://" + currentRequest.getLocalName(); if(currentRequest.getLocalPort() != 80) { baseEnvLinkURL += ":" + currentRequest.getLocalPort(); } if(!StringUtils.isEmpty(currentRequest.getContextPath())) { baseEnvLinkURL += currentRequest.getContextPath(); } return baseEnvLinkURL; }
Friday, December 12, 2014
Spring Web: Get current request and generate base URL
Just a simple way to generate application's base URL according to the environment context using current request:
Tuesday, November 4, 2014
DOS: List files recursively
To list files recursively in Windows you don't need a complex script as I thought. For example to list all .html inside subfolders you can just run simple DOS command:
dir /s/b *.html > results.txt
dir /s/b *.html > results.txt
Wednesday, October 15, 2014
Ant: adding additional JARs to classpath
I was working in a custom ATG module in which I required to extend a core ATG class. When I ran the build ant command I got compile errors because of "cannot find symbol", in other words, my dear reference class was not being recognized. It was missing! In my Eclipse project I got no errors because I had the right library included in the project's classpath.
I started looking on how to add additional JAR files to the classpath, but after digging a little bit more in the compile target referenced in the build.xml:
<target name="build" depends="echo-build-message,clean,compile,jar-classes,jar-configs,copy-to-install" />
I saw how could I add additional classpath entries using the reference id "classpath.additions". In the included common.xml file we had this:
<target name="compile"> <mkdir dir="${java.output.dir}"/> <mkdir dir="${java.src.dir}"/> <copy todir="${java.output.dir}"> <fileset dir="${java.src.dir}"> <include name="**/*.properties" /> <include name="**/*.xml" /> </fileset> </copy> <if> <isreference refid="classpath.additions" /> <then> <path id="fullClasspath"> <path refid="classpath" /> <path refid="classpath.additions" /> </path> </then> <else> <path id="fullClasspath"> <path refid="classpath" /> </path> </else> </if> <echo message="java.src.dir: ${java.src.dir}, java.output.dir: ${java.output.dir}" /> <javac srcdir="${java.src.dir}" destdir="${java.output.dir}" classpathref="fullClasspath" debug="on" includeAntRuntime="false" /> </target>
So I just added this in the build.xml:
<path id="classpath.additions"> <fileset dir="${dynamo.home}/../REST/lib"><include name="**/*.jar" /></fileset> </path>This seems like an elegant generic way for configuring classpaths in a multi module environment like ATG.
Tuesday, October 14, 2014
ATG: Nucleus tips
Get current request URI
import atg.servlet.ServletUtil; //... ServletUtil.getCurrentRequest().getRequestURI();
Define a collection of components
Java:
protected Component [] componentes; public void setComponents(Component [] components) { this.components = components; } public Component [] getComponents() { return components; }
Properties:
components=\ /path/to/some/Component,\ /path/to/another/Component
Tuesday, July 22, 2014
Spring: Integration testing under Spring Boot application context but mocking services
Use @MockBean annotation
DEPRACATED:
So I wanted to create some integration tests for an API application that uses Spring Boot. The thing is that I needed to load the same application context as the real applicationm, because Spring Boot performs a bunch of configurations for you, and these were not being loaded in a StandAlone mode.
But also I didn't want to use real services which depend upon real data. Depending on databases for your tests creates fragile tests.
After researching on the web I found this way:
This is the typical Spring-Boot entry point class.
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.EnableAutoConfiguration;
import org.springframework.context.annotation.ComponentScan;
@EnableAutoConfiguration
@ComponentScan
public class MyApplication {
public static void main(String[] args) {
SpringApplication.run(MyApplication.class, args);
}
}
A base class having the logic to init the application context.import static org.springframework.test.web.servlet.setup.MockMvcBuilders.webAppContextSetup;
import org.junit.Before;
import org.junit.runner.RunWith;
import org.mockito.MockitoAnnotations;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.SpringApplicationConfiguration;
import org.springframework.test.context.junit4.SpringJUnit4ClassRunner;
import org.springframework.test.context.web.WebAppConfiguration;
import org.springframework.test.web.servlet.MockMvc;
import org.springframework.web.context.WebApplicationContext;
@RunWith(SpringJUnit4ClassRunner.class)
@WebAppConfiguration
@SpringApplicationConfiguration(classes = MyApplication.class)
public abstract class BaseIntegrationTest {
protected MockMvc mockMvc;
@Autowired
protected WebApplicationContext wac;
@Before
public void setup() {
MockitoAnnotations.initMocks(this);
this.mockMvc = webAppContextSetup(wac).build();
}
}
An implementation class mocking a required service class.public class FooIntegrationTest extends BaseIntegrationTest {
@Configuration
public static class TestConfiguration {
@Bean
@Primary
public FooService fooService() {
return mock(FooService.class);
}
}
@Autowired
FooService mockFooService;
}
Monday, June 2, 2014
HalBuilder: First steps
My team and I are working on trying to find best Java client to consume Hal REST services. I was trying HalBuilder and took me a time to figure a few tweaks to make the simple example describe in the website to work. Perhaps, I was doing something wrong, but just in case someone is also stuck on that, here's the code:
Maven Dependencies:
<dependency> <groupId>com.theoryinpractise</groupId> <artifactId>halbuilder-api</artifactId> <version>2.2.1</version> </dependency> <dependency> <groupId>com.theoryinpractise</groupId> <artifactId>halbuilder-core</artifactId> <version>3.1.3</version> </dependency> <dependency> <groupId>com.theoryinpractise</groupId> <artifactId>halbuilder-json</artifactId> <version>3.1.3</version> </dependency> <dependency> <groupId>com.theoryinpractise</groupId> <artifactId>halbuilder-standard</artifactId> <version>3.0.1</version> </dependency> <dependency> <groupId>com.ning</groupId> <artifactId>async-http-client</artifactId> <version>1.8.9</version> </dependency>
Java code:
import java.io.IOException; import java.io.InputStreamReader; import java.util.concurrent.ExecutionException; import com.ning.http.client.AsyncHttpClient; import com.ning.http.client.Response; import com.theoryinpractise.halbuilder.api.ReadableRepresentation; import com.theoryinpractise.halbuilder.api.RepresentationFactory; import com.theoryinpractise.halbuilder.json.JsonRepresentationFactory; //http://gotohal.net/ public class TestHalBuilderAPI { public static void main(String[] args) throws InterruptedException, ExecutionException, IOException { RepresentationFactory representationFactory = new JsonRepresentationFactory(); representationFactory.withFlag(RepresentationFactory.PRETTY_PRINT); AsyncHttpClient asyncHttpClient = new AsyncHttpClient(); Response response = asyncHttpClient.prepareGet("http://gotohal.net/restbucks/api").execute().get(); InputStreamReader inputStreamReader = new InputStreamReader(response.getResponseBodyAsStream()); ReadableRepresentation representation = representationFactory.readRepresentation(inputStreamReader); String ordersLinkUrl = representation.getLinkByRel("orders").getHref(); System.out.println(ordersLinkUrl); asyncHttpClient.close(); } }
Friday, May 30, 2014
VirtualBox: Copy/Paste (Guest additions) not working on my Ubuntu VM
I have a Ubuntu VM where I do all my development. Since I installed VirtualBox and created my VM, I never was able to make copy/paste or have better graphics. It all comes with the installation of Guest Additions in the guest machine, but for some reason the installation wasn't working.
I lived with that for some time until I got time to do some more research. First I tried to run the command to enable to copy/paste:
But got an error indicating that Guest Additions was not correctly installed or setup (quite not remember well).
So then I run the setup command:
sudo /etc/init.d/vboxadd setup
And got an error:
Removing existing VirtualBox non-DKMS kernel modules ...done.
Building the VirtualBox Guest Additions kernel modules
The gcc utility was not found. If the following module compilation fails then
this could be the reason and you should try installing it.
The headers for the current running kernel were not found. If the following
module compilation fails then this could be the reason.
Building the main Guest Additions module ...fail!
(Look at /var/log/vboxadd-install.log to find out what went wrong)
Doing non-kernel setup of the Guest Additions ...done.
Stupid GCC utility was not installed! :D. Installed it:
sudo apt-get install gcc
Run again the setup and it got installed correctly. Restarted the VM and got finally my copy/paste between host and guest machines. Also my graphics acceleration.
Tuesday, May 6, 2014
ATG RQL Tips
Query any item but limit results to only one.
<query-items item-descriptor="category">ALL RANGE +1</query-items>
Remove an item.
<remove-item item-descriptor="<item-descriptor>" id="<ID>"/>
Query item where property is null/not defined
<query-items item-descriptor="product">seoTags IS NULL</query-items>
Query date ranges
Get where a property is set (not null)
<query-items item-descriptor="promotion">(NOT closenessQualifiers IS NULL) RANGE+1</query-items>
Thursday, February 13, 2014
Eclipse: JVM terminated. Exit code=13
I downloaded Eclipse for Scala and tried to open it when I got this error window message:
JVM terminated. Exit code=13
A few forums suggested a possible error in the eclipse.ini Java path but that wasn't my case. It was more related to the incompatibility of my downloaded 64-bit version of Eclipse with my default configured 32-bit Java. I have my 32-bit Java as it's the version that works for my VPN connection. I simply switched to a 64-bit Java using:
>:$ sudo update-alternatives --config java There are 3 choices for the alternative java (providing /usr/bin/java). Selection Path Priority Status ------------------------------------------------------------ 0 /usr/lib/jvm/java-7-openjdk-amd64/jre/bin/java 1071 auto mode 1 /usr/lib/jvm/java-6-openjdk-amd64/jre/bin/java 1061 manual mode 2 /usr/lib/jvm/java-7-openjdk-amd64/jre/bin/java 1071 manual mode * 3 /usr/lib/jvm/jre1.7.0_45/bin/java 1 manual mode
Tuesday, February 11, 2014
Java Core: ArrayList vs LinkedList
Performance oriented development is one key aspect of any serious developer. Sometimes we are very accustomed to some practice, or use of a specific programming language feature, that we might forget there are other options that can work better on different scenarios. This could be the case of the different collections provided by Java. In the past I used to create all my list collections using ArrayList and never wondered about the pros/cons of using alternatives like LinkedList until I read some literature explaining the best scenarios for each one.
Pros of ArrayList
1. ArrayList uses internally an array for internal storage. That makes it particularly fast for random access - get(#n).
Cons of ArrayList
1. ArrayList is slower for modification operations like add or delete elements in the beginning or middle of the collection. This is due to the need of relocate all subsequent elements one position to the right (or left in case of deletion) in order to make space to the new element.
Let's show this graphically. Let's suppose we have a list of six elements.
And we want to insert an element before the second one.

To be able to add this element, internally, the list needs to copy all elements from index 1 to 5 one position to the right.
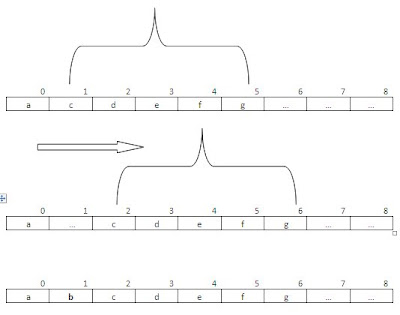
2. Similar to before described process. ArrayList has some performance downside when the internal array is completely full, and therefore has to create a bigger array and relocate all elements to new array.
Pros of LinkedList
1. LinkedList follows a different approach. It's more efficient in adding or deleting elements in the beggining or middle of the collection. If you ever programmed from scratch a list data structure, you will remember you have nodes with pointers/references to the next element.

In this case what is done to insert a new node in the middle of the list is to create a new node pointing to the next element (->c), and the before element is updated to point to the new created node (->b).
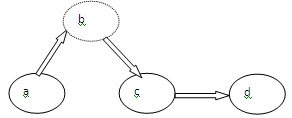

2. Given the nature of the internal structure which is not restricted to an initial size, LinkedList has no growing problems as ArrayList.
Cons of LinkedList
1. Random access to LinkedList elements are expensive, because in worst case scenarios the entire list has to be traversed to retrieve the desired element (O(n)).
We could say that we should use ArrayList if we have many random accesses. If we think our lists are going to grow unexpectedly, we should favor LinkedList. This is just one scenario. We could have both needs in which case a combination of both approaches could be use. Like using LinkedList to create the list, and then use ArrayList for read access.
Thursday, January 23, 2014
ATG Which version
This tool is very useful in ATG to find the version and location (JAR file path) of any particular class:
http://<domain>:8080/dyn/dyn/whichversion.jhtml
http://<domain>:8080/dyn/dyn/whichversion.jhtml
Subscribe to:
Posts (Atom)